本文最后更新于:2023年11月8日 中午
这篇文章主要用来总结Java在网络编程中的知识点
下面是一个Java客户端与服务端通信的样例程序
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| public class Server { public static void main(String[] args) throws IOException { ServerSocket ss = new ServerSocket(6666); System.out.println("Server start listening..."); for (; ; ) { Socket sock = ss.accept(); System.out.println("Receviced package from " + sock.getRemoteSocketAddress()); Thread t = new Handler(sock); t.start(); } } }
class Handler extends Thread { Socket sock;
public Handler(Socket sock) { this.sock = sock; }
@Override public void run() { try (InputStream input = this.sock.getInputStream()) { try (OutputStream output = this.sock.getOutputStream()) { handle(input, output); } } catch (Exception e) { try { this.sock.close(); } catch (IOException ioe) { } System.out.println("Client disconnected."); } }
private void handle(InputStream input, OutputStream output) throws IOException { var writer = new BufferedWriter(new OutputStreamWriter(output, StandardCharsets.UTF_8)); var reader = new BufferedReader(new InputStreamReader(input, StandardCharsets.UTF_8)); writer.write("hello\n"); writer.flush(); for (; ; ) { String s = reader.readLine(); if (s.equals("bye")) { writer.write("bye\n"); writer.flush(); break; } writer.write("ok: " + s + "\n"); writer.flush(); } } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| public class client { public static void main(String[] args) throws IOException { Socket sock = new Socket("localhost", 6666); try (InputStream input = sock.getInputStream()){ try(OutputStream output = sock.getOutputStream()){ handle(input, output); } } } private static void handle(InputStream input, OutputStream output) throws IOException{ var writer = new BufferedWriter(new OutputStreamWriter(output, StandardCharsets.UTF_8)); var reader = new BufferedReader(new InputStreamReader(input, StandardCharsets.UTF_8)); Scanner scanner = new Scanner(System.in); System.out.println("[server]: "+ reader.readLine()); for(;;){ System.out.print(">>>"); String s = scanner.nextLine(); writer.write(s); writer.newLine(); writer.flush(); String resp = reader.readLine(); System.out.println("<<<" + resp); if(resp.equals("bye")) break; } } }
|
实现功能为服务端与客户端相互通信,收到bye
信号后断开连接,如图所示
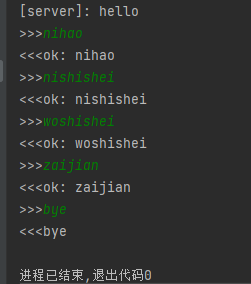